Forming Queries through the API!#
Get familiar with the tools available for querying the database. The simplest way is to use the api classes
Each class has to very useful functions
Useful Function - from_filter
#
Use the from filter function to find density profiles
# Import in our two classes to access the db
from snowexsql.api import LayerMeasurements
from datetime import datetime
# Find some density pit measurements at the Boise site in december 2019.
df = LayerMeasurements.from_filter(
type="density",
site_name="Boise River Basin",
date_less_equal=datetime(2020, 1, 1),
date_greater_equal=datetime(2019, 12, 1),
)
# Plot Example!
df.plot()
# Show off the dataframe
df
# Analysis Example - Find the bulk density
df['value'] = df['value'].astype(float)
print(df[['site_id', 'value']].groupby(by='site_id').mean())
value
site_id
Banner Open 235.500000
Banner Snotel 216.666667
Bogus Upper 260.625000
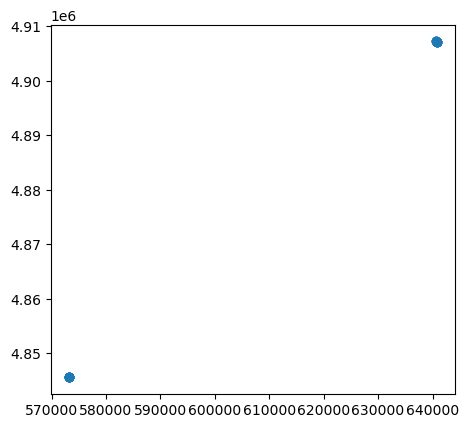
Useful Function - from_area
#
Find specific surface area within a certain distance of a pit.
# Import our api class
from snowexsql.api import LayerMeasurements
from datetime import datetime
import geopandas as gpd
# import some gis functionality
from shapely.geometry import Point
# Find some SSA measurements within a distance of a known point
pnt = Point(740820.624625,4.327326e+06)
df = LayerMeasurements.from_area(pt=pnt, crs=26912, buffer=500,
type='specific_surface_area')
# plot up the results
ax = df.plot()
# plot the site so we can see how close everything is.
site = gpd.GeoDataFrame(geometry=[pnt], crs=26912)
site.plot(ax=ax, marker='^', color='magenta')
# show off the dataframe
df
depth | site_id | pit_id | bottom_depth | comments | sample_a | sample_b | sample_c | value | flags | ... | date | time_created | time_updated | id | doi | date_accessed | instrument | type | units | observers | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
0 | 84.0 | 1C5 | COGM1C5_20200212 | None | None | None | None | None | 45.6 | None | ... | 2020-02-12 | 2024-08-15 20:03:26.508508+00:00 | None | 2407961 | https://doi.org/10.5067/SNMM6NGGKWIT | 2022-06-30 | IS3-SP-11-01F | specific_surface_area | None | Kate Hale |
1 | 79.0 | 1C5 | COGM1C5_20200212 | None | None | None | None | None | 38.2 | None | ... | 2020-02-12 | 2024-08-15 20:03:26.508508+00:00 | None | 2407962 | https://doi.org/10.5067/SNMM6NGGKWIT | 2022-06-30 | IS3-SP-11-01F | specific_surface_area | None | Kate Hale |
2 | 74.0 | 1C5 | COGM1C5_20200212 | None | None | None | None | None | 24.5 | None | ... | 2020-02-12 | 2024-08-15 20:03:26.508508+00:00 | None | 2407963 | https://doi.org/10.5067/SNMM6NGGKWIT | 2022-06-30 | IS3-SP-11-01F | specific_surface_area | None | Kate Hale |
3 | 69.0 | 1C5 | COGM1C5_20200212 | None | None | None | None | None | 23.5 | None | ... | 2020-02-12 | 2024-08-15 20:03:26.508508+00:00 | None | 2407964 | https://doi.org/10.5067/SNMM6NGGKWIT | 2022-06-30 | IS3-SP-11-01F | specific_surface_area | None | Kate Hale |
4 | 64.0 | 1C5 | COGM1C5_20200212 | None | None | None | None | None | 22.4 | None | ... | 2020-02-12 | 2024-08-15 20:03:26.508508+00:00 | None | 2407965 | https://doi.org/10.5067/SNMM6NGGKWIT | 2022-06-30 | IS3-SP-11-01F | specific_surface_area | None | Kate Hale |
... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... |
155 | 28.0 | 1C1 | COGM1C1_20200131 | None | None | None | None | None | 13.1 | None | ... | 2020-01-31 | 2024-08-15 20:03:31.924769+00:00 | None | 2410266 | https://doi.org/10.5067/SNMM6NGGKWIT | 2022-06-30 | IS3-SP-11-01F | specific_surface_area | None | Juha Lemmetyinen & Ioanna Merkouriadi |
156 | 23.0 | 1C1 | COGM1C1_20200131 | None | None | None | None | None | 10.1 | None | ... | 2020-01-31 | 2024-08-15 20:03:31.924769+00:00 | None | 2410267 | https://doi.org/10.5067/SNMM6NGGKWIT | 2022-06-30 | IS3-SP-11-01F | specific_surface_area | None | Juha Lemmetyinen & Ioanna Merkouriadi |
157 | 18.0 | 1C1 | COGM1C1_20200131 | None | None | None | None | None | 10.6 | None | ... | 2020-01-31 | 2024-08-15 20:03:31.924769+00:00 | None | 2410268 | https://doi.org/10.5067/SNMM6NGGKWIT | 2022-06-30 | IS3-SP-11-01F | specific_surface_area | None | Juha Lemmetyinen & Ioanna Merkouriadi |
158 | 13.0 | 1C1 | COGM1C1_20200131 | None | None | None | None | None | 10.5 | None | ... | 2020-01-31 | 2024-08-15 20:03:31.924769+00:00 | None | 2410269 | https://doi.org/10.5067/SNMM6NGGKWIT | 2022-06-30 | IS3-SP-11-01F | specific_surface_area | None | Juha Lemmetyinen & Ioanna Merkouriadi |
159 | 8.0 | 1C1 | COGM1C1_20200131 | None | None | None | None | None | 13.2 | None | ... | 2020-01-31 | 2024-08-15 20:03:31.924769+00:00 | None | 2410270 | https://doi.org/10.5067/SNMM6NGGKWIT | 2022-06-30 | IS3-SP-11-01F | specific_surface_area | None | Juha Lemmetyinen & Ioanna Merkouriadi |
160 rows × 29 columns
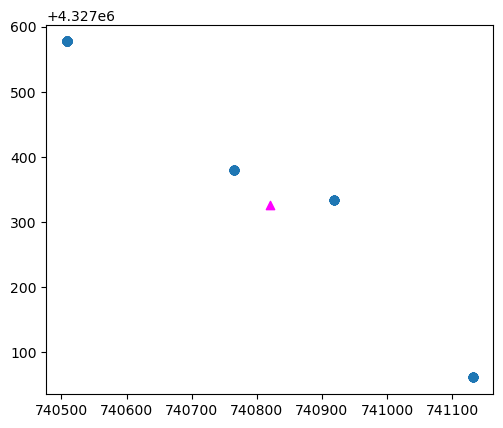
How do I know what to filter on?#
We got tools for that! Each class has a host of functions that start with all_*
these function return the unique value in that column.
all_types
- all the data types e.g. depth, swe, density…all_instruments
- all instruments available in the tableall_dates
- all dates listed in the tableall_site_names
- all the site names available in the table. e.g. Grand Mesa
from snowexsql.api import PointMeasurements
# Instantiate the class to use the properties!
measurements = PointMeasurements()
# Get the unique data names/types in the table
results = measurements.all_types
print('Available types = {}'.format(', '.join([str(r) for r in results])))
# Get the unique instrument in the table
results = measurements.all_instruments
print('\nAvailable Instruments = {}'.format(', '.join([str(r) for r in results])))
# Get the unique dates in the table
results = measurements.all_dates
print('\nAvailable Dates = {}'.format(', '.join([str(r) for r in results])))
# Get the unique site names in the table
results = measurements.all_site_names
print('\nAvailable sites = {}'.format(', '.join([str(r) for r in results])))
Available types = two_way_travel, snow_void, density, swe, depth
Available Instruments = mesa, magnaprobe, camera, pulseEkko pro 1 GHz GPR, None, Mala 1600 MHz GPR, Mala 800 MHz GPR, pulse EKKO Pro multi-polarization 1 GHz GPR, pit ruler
Available Dates = 2020-05-28, 2020-01-09, 2021-03-19, 2020-05-23, 2019-11-29, 2020-01-04, 2019-10-20, 2019-11-30, 2021-01-28, 2020-04-17, 2021-02-19, 2020-02-19, 2020-02-26, 2020-02-03, 2020-05-05, 2019-10-05, 2019-12-29, 2020-06-02, 2019-10-28, 2020-01-30, 2020-05-22, 2020-03-09, 2019-12-09, 2019-12-28, 2020-02-24, 2020-03-17, 2021-03-18, 2020-04-01, 2020-05-14, 2019-10-14, 2019-10-29, 2019-10-02, 2020-01-31, 2020-04-18, 2020-04-26, 2019-10-12, 2020-04-29, 2021-03-03, 2020-02-23, 2021-01-15, 2020-01-22, 2020-01-01, 2019-11-21, 2020-05-10, 2023-03-13, 2020-02-12, 2019-11-19, 2020-05-06, 2019-10-25, 2019-11-02, 2020-02-08, 2020-04-14, 2020-04-02, 2019-11-16, 2020-04-07, 2021-03-21, 2021-04-21, 2023-03-15, 2020-11-25, 2019-12-27, 2019-10-01, 2021-01-27, 2020-04-16, 2020-06-08, 2019-12-13, 2019-10-17, 2019-10-22, 2021-01-22, 2020-04-21, 2020-01-03, 2019-12-12, 2019-12-08, 2021-03-05, 2020-01-25, 2020-02-29, 2019-11-24, 2019-10-18, 2021-03-04, 2021-03-24, 2021-03-16, 2020-05-09, 2020-03-22, 2019-11-06, 2019-12-16, 2020-01-15, 2019-11-22, 2019-10-13, 2019-11-10, 2019-12-06, 2020-02-04, 2019-10-31, 2020-03-07, 2020-04-06, 2020-05-03, 2019-12-10, 2020-05-26, 2019-12-02, 2021-02-09, 2020-02-14, 2020-02-13, 2020-05-11, 2019-12-01, 2020-01-19, 2019-11-28, 2020-01-17, 2019-12-17, 2021-02-17, 2021-01-07, 2021-03-31, 2019-12-25, 2019-12-14, 2019-10-24, 2020-03-11, 2020-02-01, 2021-03-23, 2020-02-09, 2020-05-12, 2020-05-25, 2020-03-29, 2020-04-24, 2019-12-11, 2020-01-10, 2020-06-05, 2019-10-10, 2020-11-20, 2020-04-13, 2020-03-23, 2020-04-23, 2020-05-24, 2019-11-08, 2021-05-05, 2019-12-26, 2019-12-15, 2021-04-06, 2020-05-07, 2021-01-20, 2020-02-28, 2019-11-03, 2020-04-04, 2019-11-27, 2021-01-14, 2020-03-15, 2019-11-23, 2020-01-16, 2019-10-08, 2023-03-14, 2019-11-14, 2020-02-15, 2020-02-11, 2023-03-12, 2019-11-13, 2020-04-30, 2019-10-26, 2020-03-06, 2021-03-17, 2020-05-31, 2020-03-04, 2021-02-24, 2019-10-04, 2020-05-16, 2020-04-03, 2019-10-06, 2019-10-09, 2021-02-25, 2020-03-12, 2019-11-12, 2019-11-01, 2020-03-10, 2019-10-30, 2020-02-21, 2020-12-17, 2020-06-01, 2020-03-20, 2023-03-07, 2020-03-03, 2019-11-07, 2020-01-06, 2019-12-22, 2021-02-11, 2020-01-11, 2019-11-11, 2019-11-05, 2020-01-13, 2023-03-16, 2019-12-18, 2019-12-30, 2020-05-04, 2020-04-20, 2021-04-14, 2023-03-09, 2023-03-08, 2020-02-22, 2020-05-08, 2019-12-24, 2020-12-18, 2020-01-24, 2020-04-22, 2019-11-04, 2020-03-31, 2020-01-08, 2020-02-06, 2021-02-18, 2020-03-05, 2021-05-27, 2020-03-14, 2021-02-04, 2020-06-09, 2021-01-21, 2020-02-20, 2020-11-23, 2020-04-05, 2020-06-03, 2019-10-16, 2021-05-07, 2020-04-15, 2021-01-26, 2019-12-03, 2020-05-30, 2019-11-09, 2021-02-16, 2020-04-28, 2020-01-12, 2020-05-20, 2023-03-10, 2020-05-02, 2020-02-05, 2020-01-28, 2020-01-21, 2019-12-19, 2019-10-07, 2020-03-28, 2020-02-10, 2021-04-28, 2020-03-02, 2019-09-29, 2019-11-15, 2020-01-02, 2020-05-27, 2020-02-18, 2019-10-11, 2019-12-21, 2019-09-30, 2021-03-10, 2020-04-09, 2020-01-05, 2019-10-27, 2020-04-10, 2021-04-23, 2020-03-16, 2020-03-21, 2020-02-02, 2020-02-25, 2020-04-08, 2020-01-29, 2019-12-04, 2021-03-22, 2021-02-10, 2021-02-03, 2019-11-26, 2020-03-19, 2020-01-20, 2019-12-31, 2020-02-27, 2020-03-30, 2020-04-25, 2020-01-26, 2020-01-14, 2020-12-08, 2020-03-01, 2020-02-17, 2020-05-21, 2019-10-23, 2021-03-02, 2020-04-11, 2019-10-21, 2020-12-16, 2019-11-25, 2020-04-12, 2020-03-13, 2020-05-01, 2021-05-20, 2020-03-08, 2021-01-13, 2020-05-19, 2020-03-27, 2019-11-17, 2020-04-19, 2020-01-23, 2020-05-15, 2021-02-23, 2020-02-16, 2019-10-19, 2020-05-29, 2020-03-24, 2019-12-07, 2020-02-07, 2020-03-18, 2020-05-17, 2020-05-13, 2019-12-20, 2019-12-23, 2020-06-07, 2020-01-07, 2020-05-18, 2021-05-17, 2021-04-07, 2019-12-05, 2019-11-20, 2020-06-06, 2020-12-09, 2023-03-11, 2021-02-02, 2019-11-18, 2020-06-10, 2020-01-27, 2020-11-16, 2020-01-18, 2020-06-04, 2020-04-27, 2019-10-15, 2020-12-01, 2020-03-25, 2020-03-26, 2019-10-03, 2021-03-09
Available sites = American River Basin, Central Ag Research Center, Senator Beck, farmers-creamers, Fairbanks, None, Fraser Experimental Forest, Boise River Basin, Little Cottonwood Canyon, East River, North Slope, Jemez River, Grand Mesa, Cameron Pass, Sagehen Creek, Mammoth Lakes, Niwot Ridge
More specific filtering options#
Sometimes we need a bit more filtering to know more about what I can filter on. Questions like “What dates was the SMP used?” are a bit more complicated than “Give me all the dates for snowex”
The good news is, we have tool for that! from_unique_entries
is your friend!
# import layer measurements
from snowexsql.api import LayerMeasurements
# Query dates where SMP was used
LayerMeasurements.from_unique_entries(['date'], instrument='snowmicropen')
[datetime.date(2020, 2, 4),
datetime.date(2020, 2, 3),
datetime.date(2020, 1, 30),
datetime.date(2020, 2, 1),
datetime.date(2020, 2, 6),
datetime.date(2020, 1, 31),
datetime.date(2020, 2, 12),
datetime.date(2020, 2, 8),
datetime.date(2020, 2, 5),
datetime.date(2020, 1, 28),
datetime.date(2020, 2, 11),
datetime.date(2020, 2, 10),
datetime.date(2020, 1, 29)]
Query Nuances#
Limit size#
To avoid accidental large queries, we have added some bumper rails. By default if you ask for more than 1000 records then an error will pop up unless you explicitly say you want more.
Try This: Do a large query. Run the code block below without the limit keyword argument (“kwarg”):
# Import PointMeasurements
from snowexsql.api import PointMeasurements
# Query db using a vague filter or on a huge dataset like GPR but remove the limit kwarg
df = PointMeasurements.from_filter(type='two_way_travel', limit=100)
# Show the dataframe
df
version_number | equipment | value | latitude | longitude | northing | easting | elevation | utm_zone | geom | ... | date | time_created | time_updated | id | doi | date_accessed | instrument | type | units | observers | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
0 | None | None | 10.625499 | 39.020194 | -108.163545 | 4.322846e+06 | 745564.789805 | None | 12 | POINT (745564.790 4322846.170) | ... | 2020-02-09 | 2022-07-07 19:56:46.884180+00:00 | None | 4005062 | https://doi.org/10.5067/S5EGFLCIAB18 | 2022-07-07 | pulse EKKO Pro multi-polarization 1 GHz GPR | two_way_travel | ns | Randall Bonnell |
1 | None | None | 10.548781 | 39.020193 | -108.163545 | 4.322846e+06 | 745564.742731 | None | 12 | POINT (745564.743 4322846.082) | ... | 2020-02-09 | 2022-07-07 19:56:46.884180+00:00 | None | 4005063 | https://doi.org/10.5067/S5EGFLCIAB18 | 2022-07-07 | pulse EKKO Pro multi-polarization 1 GHz GPR | two_way_travel | ns | Randall Bonnell |
2 | None | None | 10.510421 | 39.020192 | -108.163546 | 4.322846e+06 | 745564.695657 | None | 12 | POINT (745564.696 4322845.994) | ... | 2020-02-09 | 2022-07-07 19:56:46.884180+00:00 | None | 4005064 | https://doi.org/10.5067/S5EGFLCIAB18 | 2022-07-07 | pulse EKKO Pro multi-polarization 1 GHz GPR | two_way_travel | ns | Randall Bonnell |
3 | None | None | 10.433703 | 39.020191 | -108.163547 | 4.322846e+06 | 745564.648545 | None | 12 | POINT (745564.649 4322845.906) | ... | 2020-02-09 | 2022-07-07 19:56:46.884180+00:00 | None | 4005065 | https://doi.org/10.5067/S5EGFLCIAB18 | 2022-07-07 | pulse EKKO Pro multi-polarization 1 GHz GPR | two_way_travel | ns | Randall Bonnell |
4 | None | None | 10.433703 | 39.020191 | -108.163547 | 4.322846e+06 | 745564.601508 | None | 12 | POINT (745564.602 4322845.817) | ... | 2020-02-09 | 2022-07-07 19:56:46.884180+00:00 | None | 4005066 | https://doi.org/10.5067/S5EGFLCIAB18 | 2022-07-07 | pulse EKKO Pro multi-polarization 1 GHz GPR | two_way_travel | ns | Randall Bonnell |
... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... |
95 | None | None | 11.968071 | 39.020163 | -108.163472 | 4.322843e+06 | 745571.189082 | None | 12 | POINT (745571.189 4322843.014) | ... | 2020-02-09 | 2022-07-07 19:56:46.884180+00:00 | None | 4005157 | https://doi.org/10.5067/S5EGFLCIAB18 | 2022-07-07 | pulse EKKO Pro multi-polarization 1 GHz GPR | two_way_travel | ns | Randall Bonnell |
96 | None | None | 12.044790 | 39.020164 | -108.163471 | 4.322843e+06 | 745571.285304 | None | 12 | POINT (745571.285 4322843.041) | ... | 2020-02-09 | 2022-07-07 19:56:46.884180+00:00 | None | 4005158 | https://doi.org/10.5067/S5EGFLCIAB18 | 2022-07-07 | pulse EKKO Pro multi-polarization 1 GHz GPR | two_way_travel | ns | Randall Bonnell |
97 | None | None | 12.083149 | 39.020164 | -108.163470 | 4.322843e+06 | 745571.381588 | None | 12 | POINT (745571.382 4322843.068) | ... | 2020-02-09 | 2022-07-07 19:56:46.884180+00:00 | None | 4005159 | https://doi.org/10.5067/S5EGFLCIAB18 | 2022-07-07 | pulse EKKO Pro multi-polarization 1 GHz GPR | two_way_travel | ns | Randall Bonnell |
98 | None | None | 12.159867 | 39.020164 | -108.163469 | 4.322843e+06 | 745571.477956 | None | 12 | POINT (745571.478 4322843.095) | ... | 2020-02-09 | 2022-07-07 19:56:46.884180+00:00 | None | 4005160 | https://doi.org/10.5067/S5EGFLCIAB18 | 2022-07-07 | pulse EKKO Pro multi-polarization 1 GHz GPR | two_way_travel | ns | Randall Bonnell |
99 | None | None | 12.198226 | 39.020164 | -108.163468 | 4.322843e+06 | 745571.574288 | None | 12 | POINT (745571.574 4322843.122) | ... | 2020-02-09 | 2022-07-07 19:56:46.884180+00:00 | None | 4005161 | https://doi.org/10.5067/S5EGFLCIAB18 | 2022-07-07 | pulse EKKO Pro multi-polarization 1 GHz GPR | two_way_travel | ns | Randall Bonnell |
100 rows × 23 columns
We have added this on the db to allow you to explore without accidentally pulling the entire SnowEx universe down. If you know you want a large query (defined as > 1000) then use the limit = ####
option in the from_filter
or from_area
function.
Warning - It is better to filter using other things besides the limit because the limit is not intelligent. It will simply limit the query by the order of entries that were submitted AND fits your filter. So if you encounter this then consider how to tighten up the filter.
List of Criteria#
You can use lists in your requests too!
# Import layer measurements
from snowexsql.api import LayerMeasurements
# Grab all the data that used the one of these instruments (hint hint SSA)
ssa_instruments = ["IS3-SP-15-01US", "IRIS", "IS3-SP-11-01F"]
# Query the DB (throw a limit for safety)
LayerMeasurements.from_filter(instrument=ssa_instruments, limit=100)
depth | site_id | pit_id | bottom_depth | comments | sample_a | sample_b | sample_c | value | flags | ... | date | time_created | time_updated | id | doi | date_accessed | instrument | type | units | observers | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
0 | 91.0 | 2C12 | COGM2C12_20200212 | None | None | None | None | None | 45.02 | None | ... | 2020-02-12 | 2024-08-15 20:03:26.019334+00:00 | None | 2407735 | https://doi.org/10.5067/SNMM6NGGKWIT | 2022-06-30 | IS3-SP-11-01F | reflectance | None | Kate Hale |
1 | 86.0 | 2C12 | COGM2C12_20200212 | None | None | None | None | None | 39.82 | None | ... | 2020-02-12 | 2024-08-15 20:03:26.019334+00:00 | None | 2407736 | https://doi.org/10.5067/SNMM6NGGKWIT | 2022-06-30 | IS3-SP-11-01F | reflectance | None | Kate Hale |
2 | 81.0 | 2C12 | COGM2C12_20200212 | None | None | None | None | None | 37.85 | None | ... | 2020-02-12 | 2024-08-15 20:03:26.019334+00:00 | None | 2407737 | https://doi.org/10.5067/SNMM6NGGKWIT | 2022-06-30 | IS3-SP-11-01F | reflectance | None | Kate Hale |
3 | 76.0 | 2C12 | COGM2C12_20200212 | None | None | None | None | None | 35.11 | None | ... | 2020-02-12 | 2024-08-15 20:03:26.019334+00:00 | None | 2407738 | https://doi.org/10.5067/SNMM6NGGKWIT | 2022-06-30 | IS3-SP-11-01F | reflectance | None | Kate Hale |
4 | 71.0 | 2C12 | COGM2C12_20200212 | None | None | None | None | None | 34.86 | None | ... | 2020-02-12 | 2024-08-15 20:03:26.019334+00:00 | None | 2407739 | https://doi.org/10.5067/SNMM6NGGKWIT | 2022-06-30 | IS3-SP-11-01F | reflectance | None | Kate Hale |
... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... |
95 | 72.0 | 2C13 | COGM2C13_20200212 | None | None | None | None | None | 40.5 | None | ... | 2020-02-12 | 2024-08-15 20:03:26.225144+00:00 | None | 2407830 | https://doi.org/10.5067/SNMM6NGGKWIT | 2022-06-30 | IS3-SP-11-01F | specific_surface_area | None | Kate Hale |
96 | 67.0 | 2C13 | COGM2C13_20200212 | None | None | None | None | None | 22.6 | None | ... | 2020-02-12 | 2024-08-15 20:03:26.225144+00:00 | None | 2407831 | https://doi.org/10.5067/SNMM6NGGKWIT | 2022-06-30 | IS3-SP-11-01F | specific_surface_area | None | Kate Hale |
97 | 62.0 | 2C13 | COGM2C13_20200212 | None | None | None | None | None | 26.6 | None | ... | 2020-02-12 | 2024-08-15 20:03:26.225144+00:00 | None | 2407832 | https://doi.org/10.5067/SNMM6NGGKWIT | 2022-06-30 | IS3-SP-11-01F | specific_surface_area | None | Kate Hale |
98 | 57.0 | 2C13 | COGM2C13_20200212 | None | None | None | None | None | 24.3 | None | ... | 2020-02-12 | 2024-08-15 20:03:26.225144+00:00 | None | 2407833 | https://doi.org/10.5067/SNMM6NGGKWIT | 2022-06-30 | IS3-SP-11-01F | specific_surface_area | None | Kate Hale |
99 | 52.0 | 2C13 | COGM2C13_20200212 | None | None | None | None | None | 26.0 | None | ... | 2020-02-12 | 2024-08-15 20:03:26.225144+00:00 | None | 2407834 | https://doi.org/10.5067/SNMM6NGGKWIT | 2022-06-30 | IS3-SP-11-01F | specific_surface_area | None | Kate Hale |
100 rows × 29 columns
Greater than or Less than#
Sometimes we want to isolate certain ranges of value or even dates. The greater_equal
and less_equal
terms can be added on to value
or dates
.
date_greater_equal
date_less_equal
value_greater_equal
value_less_equal
# Import the point measurements class
from snowexsql.api import PointMeasurements
# Filter values > 100 cm from the pulse ecko GPR
df = PointMeasurements.from_filter(value_greater_equal=100, type='depth', instrument='pulse EKKO Pro multi-polarization 1 GHz GPR', limit=100)
# Show off the dataframe
df
version_number | equipment | value | latitude | longitude | northing | easting | elevation | utm_zone | geom | ... | date | time_created | time_updated | id | doi | date_accessed | instrument | type | units | observers | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
0 | None | None | 100.075450 | 39.018715 | -108.177205 | 4.322645e+06 | 744387.060768 | None | 12 | POINT (744387.061 4322645.255) | ... | 2020-02-06 | 2022-07-07 19:57:21.141883+00:00 | None | 4007772 | https://doi.org/10.5067/S5EGFLCIAB18 | 2022-07-07 | pulse EKKO Pro multi-polarization 1 GHz GPR | depth | cm | Randall Bonnell |
1 | None | None | 100.575830 | 39.018713 | -108.177204 | 4.322645e+06 | 744387.159823 | None | 12 | POINT (744387.160 4322644.972) | ... | 2020-02-06 | 2022-07-07 19:57:21.141883+00:00 | None | 4007773 | https://doi.org/10.5067/S5EGFLCIAB18 | 2022-07-07 | pulse EKKO Pro multi-polarization 1 GHz GPR | depth | cm | Randall Bonnell |
2 | None | None | 100.575830 | 39.018710 | -108.177203 | 4.322645e+06 | 744387.259312 | None | 12 | POINT (744387.259 4322644.689) | ... | 2020-02-06 | 2022-07-07 19:57:21.141883+00:00 | None | 4007774 | https://doi.org/10.5067/S5EGFLCIAB18 | 2022-07-07 | pulse EKKO Pro multi-polarization 1 GHz GPR | depth | cm | Randall Bonnell |
3 | None | None | 100.575830 | 39.018708 | -108.177202 | 4.322644e+06 | 744387.359537 | None | 12 | POINT (744387.360 4322644.406) | ... | 2020-02-06 | 2022-07-07 19:57:21.141883+00:00 | None | 4007775 | https://doi.org/10.5067/S5EGFLCIAB18 | 2022-07-07 | pulse EKKO Pro multi-polarization 1 GHz GPR | depth | cm | Randall Bonnell |
4 | None | None | 100.075450 | 39.018705 | -108.177201 | 4.322644e+06 | 744387.459761 | None | 12 | POINT (744387.460 4322644.124) | ... | 2020-02-06 | 2022-07-07 19:57:21.141883+00:00 | None | 4007776 | https://doi.org/10.5067/S5EGFLCIAB18 | 2022-07-07 | pulse EKKO Pro multi-polarization 1 GHz GPR | depth | cm | Randall Bonnell |
... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... |
95 | None | None | 103.578097 | 39.018577 | -108.176503 | 4.322632e+06 | 744448.372728 | None | 12 | POINT (744448.373 4322631.820) | ... | 2020-02-06 | 2022-07-07 19:57:21.141883+00:00 | None | 4008003 | https://doi.org/10.5067/S5EGFLCIAB18 | 2022-07-07 | pulse EKKO Pro multi-polarization 1 GHz GPR | depth | cm | Randall Bonnell |
96 | None | None | 103.578097 | 39.018578 | -108.176499 | 4.322632e+06 | 744448.664264 | None | 12 | POINT (744448.664 4322631.891) | ... | 2020-02-06 | 2022-07-07 19:57:21.141883+00:00 | None | 4008004 | https://doi.org/10.5067/S5EGFLCIAB18 | 2022-07-07 | pulse EKKO Pro multi-polarization 1 GHz GPR | depth | cm | Randall Bonnell |
97 | None | None | 103.077717 | 39.018578 | -108.176496 | 4.322632e+06 | 744448.955799 | None | 12 | POINT (744448.956 4322631.962) | ... | 2020-02-06 | 2022-07-07 19:57:21.141883+00:00 | None | 4008005 | https://doi.org/10.5067/S5EGFLCIAB18 | 2022-07-07 | pulse EKKO Pro multi-polarization 1 GHz GPR | depth | cm | Randall Bonnell |
98 | None | None | 100.075450 | 39.018581 | -108.176482 | 4.322632e+06 | 744450.096586 | None | 12 | POINT (744450.097 4322632.327) | ... | 2020-02-06 | 2022-07-07 19:57:21.141883+00:00 | None | 4008009 | https://doi.org/10.5067/S5EGFLCIAB18 | 2022-07-07 | pulse EKKO Pro multi-polarization 1 GHz GPR | depth | cm | Randall Bonnell |
99 | None | None | 100.575830 | 39.018606 | -108.176377 | 4.322635e+06 | 744459.151034 | None | 12 | POINT (744459.151 4322635.383) | ... | 2020-02-06 | 2022-07-07 19:57:21.141883+00:00 | None | 4008041 | https://doi.org/10.5067/S5EGFLCIAB18 | 2022-07-07 | pulse EKKO Pro multi-polarization 1 GHz GPR | depth | cm | Randall Bonnell |
100 rows × 23 columns
Recap#
You just came in contact with the new API tools. We can use each API class to pull from specific tables and filter the data. You should know:
How to build queries using
from_filter
,from_area
,from_unique_entries
Determine what values to filter on
Manage the limit error
Filtering on greater and less than
If you don’t feel comfortable with these, you are probably not alone, let’s discuss it!