Exercise: Visualize a Manual Depth Spiral#
During the SnowEx campaigns a TON of manual snow depths were collected, past surveys for hackweek showed an overhelming interest in the manual snow depths dataset. This tutorial shows how easy it is to get at that data in the database while learning how to build queries
Goal: Visualize a small subset of snow depth, ideally a full spiral (mostly cause they are cool!)
Approach:
Determine the necessary details for isolating manual depths
Find a pit where many spirals were done.
Buffer on the pit location and grab all manual snow depths
Process#
from snowexsql.api import LayerMeasurements
data_type = 'depth'
Step 1: Find a pit of interest#
# Pick the first one we find
site_id = LayerMeasurements().all_site_ids[0]
# Query the database, we only need one point to get a site id and its geometry
site_df = LayerMeasurements.from_filter(site_id=site_id, limit=1)
# Print it out
site_df
depth | site_id | pit_id | bottom_depth | comments | sample_a | sample_b | sample_c | value | flags | ... | date | time_created | time_updated | id | doi | date_accessed | instrument | type | units | observers | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
0 | 66.0 | 1N1 | COGM1N1_20200208 | None | None | None | None | None | 47.94 | None | ... | 2020-02-08 | 2024-08-15 20:03:40.149956+00:00 | None | 2413819 | https://doi.org/10.5067/SNMM6NGGKWIT | 2022-06-30 | IS3-SP-15-01US | reflectance | None | Kehan Yang |
1 rows × 29 columns
Step 2: Collect Snow Depths#
# We import the points measurements because snow depths is a single value at single location and date
from snowexsql.api import PointMeasurements
# Filter the results to within 100m within the point from our pit
df = PointMeasurements.from_area(pt=site_df.geometry[0], type=data_type, buffer=200)
df
version_number | equipment | value | latitude | longitude | northing | easting | elevation | utm_zone | geom | ... | date | time_created | time_updated | id | doi | date_accessed | instrument | type | units | observers | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
0 | 1 | CRREL_C | 86.0 | 39.03632 | -108.22103 | 4.324482e+06 | 740532.506729 | 3028.7 | 12 | POINT (740532.507 4324482.477) | ... | 2020-01-28 | 2022-06-30 22:56:52.635035+00:00 | None | 5597 | https://doi.org/10.5067/9IA978JIACAR | 2022-06-30 | magnaprobe | depth | cm | None |
1 | 1 | CRREL_C | 78.0 | 39.03631 | -108.22100 | 4.324481e+06 | 740535.137724 | 3027.6 | 12 | POINT (740535.138 4324481.446) | ... | 2020-01-28 | 2022-06-30 22:56:52.635035+00:00 | None | 5598 | https://doi.org/10.5067/9IA978JIACAR | 2022-06-30 | magnaprobe | depth | cm | None |
2 | 1 | CRREL_C | 71.0 | 39.03631 | -108.22096 | 4.324482e+06 | 740538.600473 | 3028.8 | 12 | POINT (740538.600 4324481.552) | ... | 2020-01-28 | 2022-06-30 22:56:52.635035+00:00 | None | 5599 | https://doi.org/10.5067/9IA978JIACAR | 2022-06-30 | magnaprobe | depth | cm | None |
3 | 1 | CRREL_C | 78.0 | 39.03631 | -108.22093 | 4.324482e+06 | 740541.197535 | 3028.4 | 12 | POINT (740541.198 4324481.631) | ... | 2020-01-28 | 2022-06-30 22:56:52.635035+00:00 | None | 5600 | https://doi.org/10.5067/9IA978JIACAR | 2022-06-30 | magnaprobe | depth | cm | None |
4 | 1 | CRREL_C | 95.0 | 39.03631 | -108.22091 | 4.324482e+06 | 740542.928909 | 3028.4 | 12 | POINT (740542.929 4324481.684) | ... | 2020-01-28 | 2022-06-30 22:56:52.635035+00:00 | None | 5601 | https://doi.org/10.5067/9IA978JIACAR | 2022-06-30 | magnaprobe | depth | cm | None |
... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... |
362 | 1 | CRREL_A | 98.0 | 39.03458 | -108.21989 | 4.324292e+06 | 740637.101899 | 3032.6 | 12 | POINT (740637.102 4324292.356) | ... | 2020-02-11 | 2022-06-30 22:56:52.635035+00:00 | None | 33920 | https://doi.org/10.5067/9IA978JIACAR | 2022-06-30 | magnaprobe | depth | cm | None |
363 | 1 | CRREL_A | 92.0 | 39.03455 | -108.21990 | 4.324289e+06 | 740636.338030 | 3034.3 | 12 | POINT (740636.338 4324289.000) | ... | 2020-02-11 | 2022-06-30 22:56:52.635035+00:00 | None | 33921 | https://doi.org/10.5067/9IA978JIACAR | 2022-06-30 | magnaprobe | depth | cm | None |
364 | 1 | CRREL_A | 80.0 | 39.03454 | -108.21992 | 4.324288e+06 | 740634.640558 | 3034.6 | 12 | POINT (740634.641 4324287.837) | ... | 2020-02-11 | 2022-06-30 22:56:52.635035+00:00 | None | 33922 | https://doi.org/10.5067/9IA978JIACAR | 2022-06-30 | magnaprobe | depth | cm | None |
365 | 1 | CRREL_A | 93.0 | 39.03452 | -108.21995 | 4.324286e+06 | 740632.111323 | 3034.2 | 12 | POINT (740632.111 4324285.537) | ... | 2020-02-11 | 2022-06-30 22:56:52.635035+00:00 | None | 33923 | https://doi.org/10.5067/9IA978JIACAR | 2022-06-30 | magnaprobe | depth | cm | None |
366 | 1 | CRREL_A | 98.0 | 39.03448 | -108.21995 | 4.324281e+06 | 740632.247106 | 3034.9 | 12 | POINT (740632.247 4324281.097) | ... | 2020-02-11 | 2022-06-30 22:56:52.635035+00:00 | None | 33924 | https://doi.org/10.5067/9IA978JIACAR | 2022-06-30 | magnaprobe | depth | cm | None |
367 rows × 23 columns
Step 3: Plot it!#
# Get the Matplotlib Axes object from the dataframe object, color the points by snow depth value
ax = df.plot(column='value', legend=True, cmap='PuBu')
site_df.plot(ax=ax, marker='^', color='m')
# Use non-scientific notation for x and y ticks
ax.ticklabel_format(style='plain', useOffset=False)
# Set the various plots x/y labels and title.
ax.set_title(f'{len(df.index)} Manual Snow depths collected at {site_id}')
ax.set_xlabel('Easting [m]')
ax.set_ylabel('Northing [m]')
Text(121.05281821225769, 0.5, 'Northing [m]')
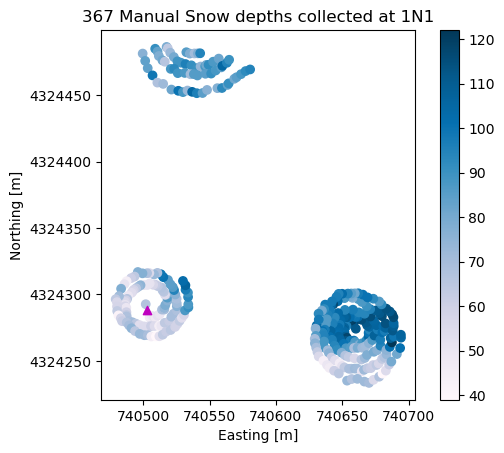
Try This:
A. Go back and add a filter to reduce to just one spiral. What would you change to reduce this?
B. Try to filtering to add more spirals. What happens?
Recap#
You just plotted snow depths and reduce the scope of the data by using from_area
on it
You should know:
Manual depths are neat.
filter using from area is pretty slick.
We can use LayerMeasurements to get site details easily.
If you don’t feel comfortable with these, you are probably not alone, let’s discuss it!